Notification Center
If the host application want to implement one notification center inside itself, can implement the listener provide by Siprocal SDK.
Step 1 : NotificationDataListener
In the class that extend of Application class implement the listener NotificationDataListener
like this :
public class MainApplication extends Application implements NotificationDataListener {
@Override
public void onCreate() {
super.onCreate();
...
// SDK initizalization
...
SiprocalSDK.addNotificationDataListener(this);
}
@Override
public void onTerminate() {
SiprocalSDK.removeNotificationDataListener();
super.onTerminate();
}
@Override
public void onNotificationDataListener(@NonNull NotificationData notificationData) {
//use the notification data received
}
}
class MainApplication : Application(), NotificationDataListener {
override fun onCreate() {
super.onCreate()
...
// SDK initizalization
...
SiprocalSDK.addNotificationDataListener(this)
}
override fun onTerminate() {
SiprocalSDK.removeNotificationDataListener()
super.onTerminate()
}
override fun onNotificationDataListener(notificationData: NotificationData) {
//use the notification data received
}
}
In the previous code we do the next:
-
In onCreate() method with the line SiprocalSDK.addNotificationDataListener(this) we
subscribe to the listener for can receive the notification data when Siprocal SDK receive one
notification -
In onTerminate() method witht he line SiprocalSDK.removeNotificationDataListener()
we remove the listener before the app going to dead to avoid memory leak. -
onNotificationDataListener is the method with the app is going to receive the notification data in
the param notificationData.
NotificationData this object contain the next properties:
- adId(Long) : id used by SDK to identify the Ad
- actionUrl(String): Url for CTA include in the Ad
- actionType(Int): Number integer to identify the type of CTA,
1 : mean actionUrl could be a URL to be open in the browser (https://siprocal.com) or open the
call app (telf:123),
2 : a deeplink (app://activity_sample),
3 : open a app, actionUrl contain package name (com.myapp.sample) - category(String): To identify the category of the Ad, this is customized by the customer using
the platform when create the campaign. - actionId(String): One identifier is related to the category, this is customized by the
customer using the platform when create the campaign - createdAt(Long): Value of type long that could be converted in format date for example:
1704088800000 -> Mon Jan 01 2024 06:00:00. Represent the time in the Ad was created - startedAt(Long): Value of type long that could be converted in format date for example:
1704088800000 -> Mon Jan 01 2024 06:00:00. Represent the time in the Ad was created - finalizedAt(Long): Value of type long that could be converted in format date for example:
1704607200000 -> Sun Jan 07 2024 06:00:00. Represent the time when Ad is expired - notificationTitle(String): Title text of the notification
- notificationDescription(String): Text of description of notification
- icon(String): Url of the Icon of notification
- bigImage(String): Url of the large image of notification (this only apply for rich image
format notification)
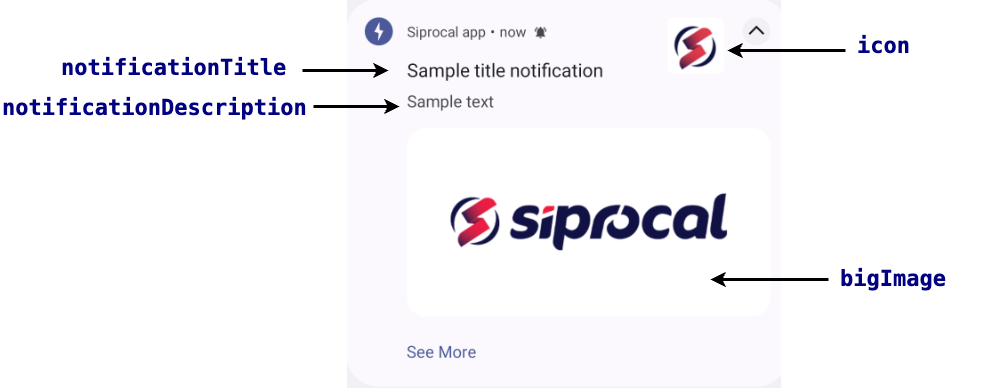
Step 2 : NotificationEventListener
In case also want to receive events from the notification Siprocal SDK provide other listener for receive event like VIEWED, CLOSED_NOTIFICATION, CLICK, only need to implement the listener NotificationEventListener similar how we implement NotificationDataListener
public class MainApplication extends Application implements NotificationDataListener, NotificationEventListener {
@Override
public void onCreate() {
super.onCreate();
...
// SDK initizalization
...
SiprocalSDK.addNotificationDataListener(this);
SiprocalSDK.addNotificationEventListener(this)
}
@Override
public void onTerminate() {
SiprocalSDK.removeNotificationDataListener();
SiprocalSDK.removeNotificationEventListener()
super.onTerminate();
}
@Override
public void onNotificationDataListener(@NonNull NotificationData notificationData) {
//use the notification data received
}
@Override
public void onNotificationEventListener(@NonNull Long adId, @NonNull NotificationEventType.Type notificationEventType) {
//get the event from notificationEventType param
}
}
class MainApplication : Application(), NotificationDataListener, NotificationEventListener {
override fun onCreate() {
super.onCreate()
...
// SDK initizalization
...
SiprocalSDK.addNotificationDataListener(this)
SiprocalSDK.addNotificationEventListener(this)
}
override fun onTerminate() {
SiprocalSDK.removeNotificationDataListener()
SiprocalSDK.removeNotificationEventListener()
super.onTerminate()
}
override fun onNotificationDataListener(notificationData: NotificationData) {
//use the notification data received
}
override fun onNotificationEventListener(
adId: Long, notificationEventType: NotificationEventType.Type
) {
//get the event from notificationEventType param
}
}
In the override method onNotificationEventListener, we get how first parameter the AdId of type Long use by Siprocal Sdk to identify the events for the Ad, and as second parameter the event that could be:
- VIEWED: Notification was shown.
- CLOSED_NOTIFICATION: The notification is close.
- CLICK: The notification is clicked
Step 3: Send event
If the host app wishes to synchronize the actions between notification center and device's
notification center, the method below should be used to send informations about campaigns' events to
Siprocal SDK
SiprocalSDK.sendNotificationEventFromHostApp(getApplicationContext(),adId,NotificationEventType.Type.CLICK);
SiprocalSDK.sendNotificationEventFromHostApp(applicationContext, adId, NotificationEventType.Type.CLICK)
- Context: application context
- adId: adId of type Long, used by Siprocal SDK to identify the Ad. This datum coming in
NotificationData object received with help of NotificationDataListener - notificationEventType: This is the event that was be send to SDK, for this we use the class NotificationEventType.Type
Updated 9 months ago